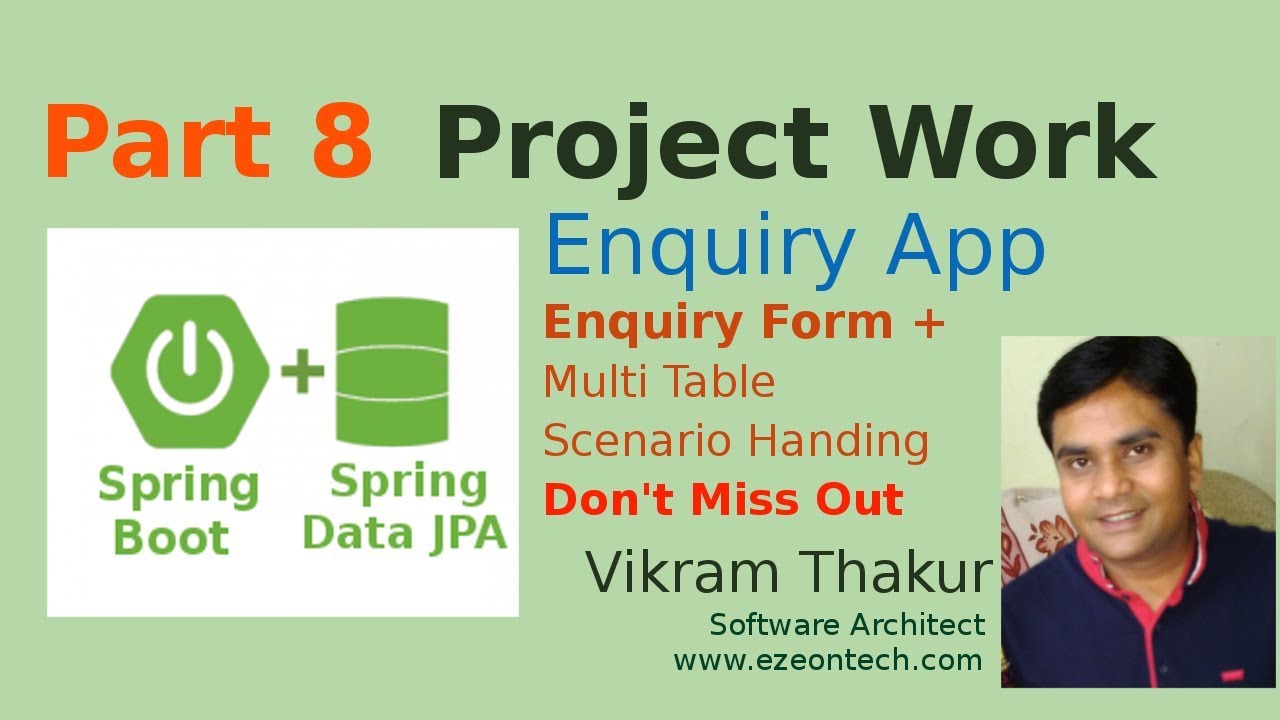
PURPOSE:
This video covers Adding Enquiry. Its using advanced form handing approach and finally processing the form data using Spring Data JPA Hibernate internally with @Transactional approach.
Enquiry Entity is a major entity of this project and whole functionality is designed around this entity. Moremover this single form covers 7 entities : Enqury, EnquirySource, EnquiryCourse, Contact, Address, Institute and Course. The entity Followup is not used in this video.
This form uses EnquiryCommand object to bind form properties used in Thymeleaf Template page. I tried to reuse most of the Enquiry entity properties by wrapping Enquiry into EnquiryCommand using Has-A relation.
The form using reference data : Institute List, Courses and Enquiry Source.
Courses are loaded in form based on Institute Selection. Here jQuery AJAX call is used to fetch courses and fees for selected institute. The form has Committed Fees Option which is filled when user checks Courses using small JavaScript code.
The example covers Spring Data JPA Query Methods like findByName(String name), findById(Long id), "public List of Course findCourseListByInstitute_instituteId(Long instituteId)" etc. These inbuilt convention saves many lines of code. Thanks to Spring Data API which has automated such common use-cases.
What is Query Method: Query methods are methods that find information from the database and are declared on the repository interface. Its a unique feature of Spring Data JPA.
If you are not fan of Hibernate/JPA or Any such ORM technology. I suggest you please try Spring Data API its saves may lines of code and save cost. Also high productive technology.
Finally @Transaction is used to manage the data integrity as the form data is divided in multiple tables. If all okay then transaction is committed. Else transaction will be automatically rolled back. This Transaction is also a great feature offered by Spring Framework.
SOURCE CODE REFERENCE:
1. EnquiryController Mappings CODE:
@GetMapping("/")
public String index(Model m) {
m.addAttribute("cmd", new EnquiryCommand());
return "/index"; //index.html page
}
@GetMapping("/save-enquiry")
public String save(@ModelAttribute EnquiryCommand cmd) {
commonService.saveEnquiry(cmd);
return "redirect:/enq-list";
}
2. REFERENCE FORM CODE:
@ModelAttribute("instituteList")
public List of Institute getInstList() {
return instituteRepository.getInstCostomList();
}
@ModelAttribute("sourceList")
public List of EnquirySource getSourceList() {
return enquirySourceRepository.findAll();
}
@GetMapping("/get-courses")
@ResponseBody
public List of Course getCoursesByInstituteId(@RequestParam Long instId) {
return courseRepository.findCourseListByInstitute_instituteId(instId);
}
3. SAVE FORM HANDING CODE:
@Transactional
public void saveEnquiry(EnquiryCommand cmd) {
Address a = new Address(cmd.getAddress());
addressRepository.save(a);
Contact c = cmd.getEnquiry().getContact();
c.setPermanentAddress(a);
contactRepository.save(c);
Enquiry e = cmd.getEnquiry();
Date d = new Date();
e.setDoe(d);
e.setLastUpdate(d);
e.setInstitute(instituteRepository.getOne(e.getInstitute().getInstituteId()));
e.setEnquirySource(enquirySourceRepository.getOne(e.getEnquirySource().getEnquirySourceId()));
enquiryRepository.save(e);
for (Long courseId : cmd.getCourses()) {
EnquiryCourse ec = new EnquiryCourse();
ec.setCourse(courseRepository.getOne(courseId));
ec.setEnquiry(e);
enquiryCourseRepository.save(ec);
}
}
TECHNOLOGY USED:
Java 8, Spring Boot, Spring Data JPA, MySQL Database, NetBeans IDE, Hibernate JPA Implementation, Maven, MySQL Workbench, JSON, MicroServices.
PREVIOUS VIDEO IN CASE YOU MISSED (Spring Data JPA | Spring Boot | Project Work - Course Save and List | Class & Map Projection) :
0 Comments